I have a teeny tiny pet peeve with dialogue boxes. Er, not dialog boxes — dialogue boxes, the ones in video games with scrolling lines of dialogue.
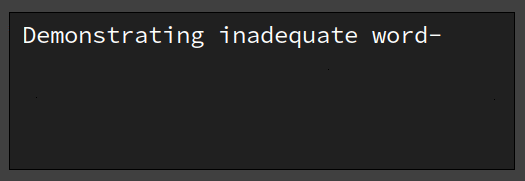
I recently wrote a dialogue box, and I saw a game that made this mistake, so here’s a post about it.
Obvious, simple, but wrong
Here’s a live example of the above animation. (You can double-click on any of these to restart them.)
And the code responsible. I wrote this in the form of a fairly generic update()
function, rather than in terms of requestAnimationFrame
, to minimize the DOM-specific stuff. All the JS in this post is vanilla DOM.
1"use strict";
2var TEXT = "Demonstrating inadequate word-wrapping functionality necessitates conspicuously verbose representative scripture.";
3var SPEED = 8; // characters per second
4
5// Number of characters currently visible
6var cursor = 0;
7// Elapsed time * SPEED, so every time this value increases by
8// 1, one more character should be displayed
9var timer = 0;
10function update(dt) {
11 timer += dt * SPEED;
12 while (timer >= 1 && cursor < TEXT.length) {
13 // Don't count spaces as characters
14 if (TEXT.charAt(cursor) != " ") {
15 timer -= 1;
16 }
17
18 cursor += 1;
19 }
20
21 var el = document.getElementById('target');
22 el.textContent = TEXT.substr(0, cursor);
23
24 // Stop updating once we run out of text
25 if (cursor >= TEXT.length) {
26 return false;
27 }
28}
If you’ve ever written dialogue handling code, this shouldn’t be too surprising. Multiplying dt
(seconds) by SPEED
(characters per second) produces a number of characters, so whenever timer
is at least 1, another character should be displayed. Spaces are counted as “free”; otherwise, the scrolling would seem to pause between words.
(The above code has a bug, as does most “string” manipulation code in JavaScript: it cuts astral plane characters in half, briefly displaying garbage. Fixing this is left as an exercise.)
The problem, of course, is that the resulting text looks like this on successive frames, where the |
s mark the edges of the box:
|Demonstrating inadequate word-wra |
|Demonstrating inadequate word-wrap|
|Demonstrating inadequate |
|word-wrapp |
And so on. The renderer has no way of knowing that “word-wrap” is only part of a longer word, so it merrily puts everything on one line. The player then sees half a word abruptly jump to a new line, and judges you harshly for it.
Depending on your environment, you can solve this one of two ways, or not-solve it a third way.
Render everything, but only draw some of it
This works well in browser-based games, where you have a comically powerful text rendering engine at your fingertips. In graphics-oriented engines that don’t offer any text rendering beyond “print this text to the screen somewhere”, this approach may not be practical.
The idea is to always “draw” the entire phrase, but implement scrolling by making it partially invisible. Consider this HTML:
1<span class="visible">Demonstra</span><span class="invisible">ting</span>
Even though the word is split across two tags, the browser must still treat it as a single word, because there’s no space anywhere. So the phrase will be word-wrapped correctly from the beginning, and the problem is solved.
You could implement this with only two <span>
s, as above, but that forces the browser to reflow the text every single frame. It probably doesn’t make a visible difference, but I prefer to wrap each character in its own <span>
and simply make them visible one at a time. As a minor bonus, you can put whitespace in the same <span>
as the preceding letter, and you won’t have to worry about it within your update loop.
Also, if your text contains formatting — i.e., more HTML — then one <span>
per character is much simpler to deal with. (Dealing with it is left as an exercise.)
Here it is live:
1"use strict";
2var TEXT = "Demonstrating inadequate word-wrapping functionality necessitates conspicuously verbose representative scripture.";
3var SPEED = 8; // characters per second
4
5// The first invisible letter <span>
6var next_letter = null;
7
8function init() {
9 var el = document.getElementById('target');
10
11 // Setup: populate the element with the entire phrase,
12 // split into characters, each wrapped in a <span>
13 var i = 0;
14 while (i < TEXT.length) {
15 var span = document.createElement('span');
16 span.classList.add('js-invisible');
17 span.textContent = TEXT.charAt(i);
18 el.appendChild(span);
19 i += 1;
20
21 // Also include any following whitespace
22 var ch;
23 while ((ch = TEXT.charAt(i)) == " ") {
24 span.textContent += ch;
25 i += 1;
26 }
27 }
28
29 next_letter = el.firstChild;
30}
31
32// Elapsed time * SPEED, so every time this value increases by
33// 1, one more character should be displayed
34var timer = 0;
35function update(dt) {
36 timer += dt * SPEED;
37 while (timer >= 1) {
38 timer -= 1;
39 next_letter.classList.remove('js-invisible');
40 next_letter = next_letter.nextSibling;
41
42 // Stop updating once we run out of text
43 if (next_letter == null) {
44 return false;
45 }
46 }
47}
I added an init()
function (called from a load
handler, not shown here) to do the setup and split the string into a series of <span>
s. (If you wanted to be especially clever, you could use the DocumentFragment
API here, but I’m not sure it’d make a real difference.) The main loop becomes much simpler: rather than counting characters, it can use the DOM tree API and hop from one <span>
to the next with .nextSibling
. Once you hit null
, you’ve run out of characters, so you’re done.
The CSS is merely:
1.js-invisible {
2 visibility: hidden;
3}
Be sure to use visibility: hidden;
here and NOT display: none;
! The latter tells the browser to ignore the hidden characters while rendering, which defeats the whole purpose of having them.
Hard wrap ahead of time
The other fix is to keep drawing one character at a time, but split the phrase into lines once ahead of time.
DO NOT use your programming language’s standard library to do this. DO NOT just Google for code that does this. You will get something that word wraps based on number of characters without taking the font into account, and the results will be wrong.
DO NOT fudge it by guessing the width of the “average” character. You will hit edge cases, and they will look ridiculous.
Find something in your graphics library to do this for you. For example, LÖVE has the poorly-named Font:getWrap
: it takes a string of text and a width, and it returns a set of wrapped strings, one per line.
(Of course, if your font is monospace and will always be monospace, feel free to do naïve word-wrap.)
Font-aware word-wrapping is surprisingly difficult in JavaScript, even though it’s sitting on top of a glorified text renderer, so in the following example I’ve totally fudged it. It may not work the same way on your screen that it does on mine, which is why you shouldn’t be fudging it.
1"use strict";
2var TEXT = "Demonstrating inadequate word-wrapping functionality necessitates conspicuously verbose representative scripture.";
3var SPEED = 8; // characters per second
4
5function init() {
6 // This is hard in JavaScript, so just pretend there's
7 // an API to do it for us
8 //var lines = magical_word_wrap_api(TEXT);
9 var lines = [
10 "Demonstrating inadequate",
11 "word-wrapping functionality",
12 "necessitates conspicuously verbose",
13 "representative scripture."
14 ];
15 TEXT = lines.join('\n');
16}
17
18// Number of characters currently visible
19var cursor = 0;
20// Elapsed time * SPEED, so every time this value increases by
21// 1, one more character should be displayed
22var timer = 0;
23function update(dt) {
24 timer += dt * SPEED;
25 while (timer >= 1 && cursor < TEXT.length) {
26 // Don't count spaces as characters
27 if (TEXT.charAt(cursor).match(/\S/)) {
28 timer -= 1;
29 }
30
31 cursor += 1;
32 }
33
34 var el = document.getElementById('target');
35 el.textContent = TEXT.substr(0, cursor);
36
37 // Stop updating once we run out of text
38 if (cursor >= TEXT.length) {
39 return false;
40 }
41}
This code is fairly similar to the original, since the basic idea is the same. All I did was add the init()
step and change the space code to also skip over newlines.
And, hm, that’s all there is to it, really.
The desperate approach
Maybe you don’t have a fancy text rendering engine, and you don’t have any way to correctly break the text, and you’re dead set on using a proportional font.
At this point I would be questioning some of the decisions that had brought me to this point in my life, but you do still have one final recourse. The classic solution, dating back decades. Pokémon did it. Come to think of it, Pokémon might still do it.
What you do is: manually include line breaks in your dialogue. All of it. Everywhere.
That is, instead of this:
1var TEXT = "Demonstrating inadequate word-wrapping functionality necessitates conspicuously verbose representative scripture.";
You will need to literally have this:
1var TEXT = "Demonstrating inadequate\nword-wrapping functionality\nnecessitates conspicuously verbose\nrepresentative scripture.";
Have fun.
Yeah
I hope at least one person reads this and goes to fix the word-wrapping in their scrolling dialogue. I’ll have made the world a slightly better place. 🌈